Jest can be very helpful framework when testing your application. Until it isn’t. Sometimes it gets in the way, and to configure it properly can be quite tricky.
Problematic use case
So, you have just learned that it is better to use specific imports instead of the { destruct } syntatx. Why? Because it makes smaller size of the bundle. So, for example when you are using lodash, you want to use import cloneDeep from 'lodash-es/cloneDeep';
instead of import { cloneDeep } from 'lodash-es';
.
// This references the whole lodash-es npm package, and then pickups the cloneDeep function from it.
// Because of referencing the whole package, it increases the bundle size.
import { cloneDeep } from 'lodash-es';
// This only references the specific lodash function, and its dependencies.
// So the resulting bundle is smaller.
import cloneDeep from 'lodash-es/cloneDeep';
It is even recommended by Lodash authors.
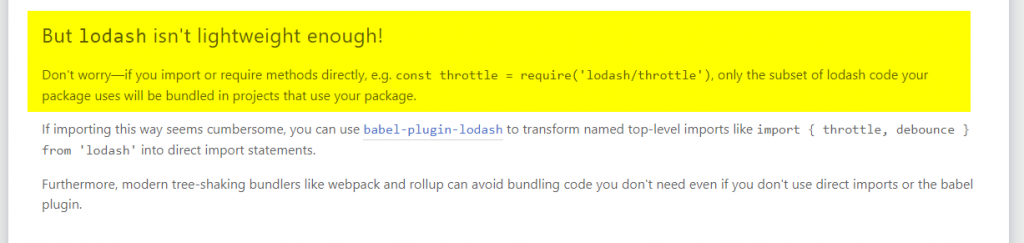
Jest vs ES modules
As the name suggests, lodash-es uses ES6 modules syntax. Jest does not like it, and the unit tests will fail with syntax error. The error message will be something like this SyntaxError: Cannot use import statement outside a module
. Not very helpful message, but try to search it, DuckDuckGo will yield ton of results.
How to fix it
Update your jest.config.js
file like this:
// jest.config.js
module.exports = {
// ... rest of Jest configuration is not shown
transform: {},
transformIgnorePatterns: ['/!node_modules\\/lodash-es/*'],
moduleNameMapper: {
'^lodash-es$': 'lodash',
'^lodash-es/cloneDeep$': 'lodash/cloneDeep',
'^lodash-es/anyOtherLodashModule$': 'lodash/anyOtherLodashModule',
},
}
Happy coding!